Table Of Contents
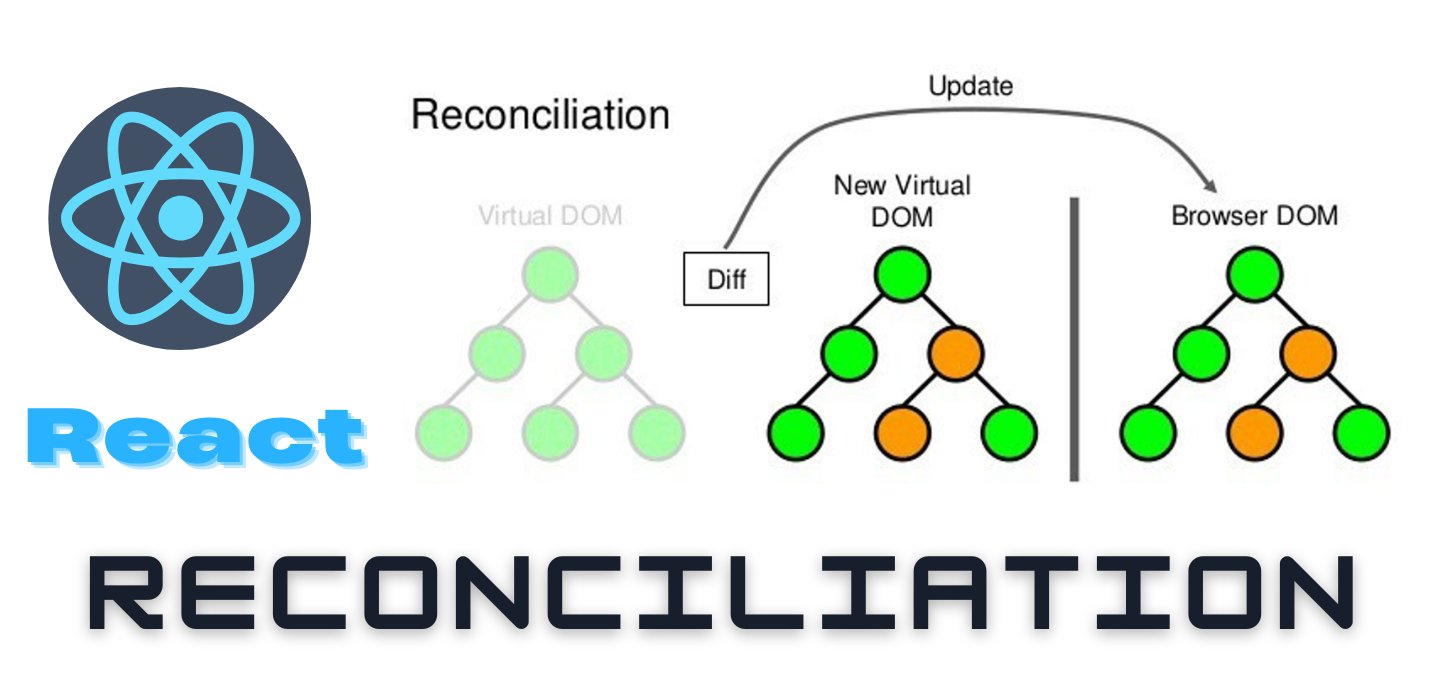
What Is Reconciliation in React, and How Does It Optimize Performance
Reconciliation is the process React uses to efficiently update the UI while minimizing unnecessary DOM operations. Instead of re-rendering everything when state or props change, React intelligently determines what has changed and updates only those specific parts of the DOM.
This is one of React’s biggest advantages, ensuring applications stay fast, responsive, and efficient—even as UIs grow in complexity. But how exactly does React achieve this? Let’s go deeper into the behind-the-scenes optimizations that make reconciliation a crucial part of React’s architecture.
Why React Needs Reconciliation
In a React application, the UI is constantly evolving. Every time a user interacts with the app—clicking a button, submitting a form, updating input fields—React needs to reflect those changes in the browser.
However, the real DOM is slow when handling frequent updates because each modification triggers:
- Style recalculations across elements
- Layout reflows to reposition affected nodes
- Repaints to redraw parts of the UI
If React were to update the entire UI on every state change, performance would suffer. Instead, React only updates what has changed, thanks to reconciliation.
How Reconciliation Works in React
React Updates the Virtual DOM
Instead of modifying the real DOM directly, React first updates an in-memory representation called the Virtual DOM. This is a lightweight copy of the actual UI, stored as JavaScript objects.
React Compares the New and Previous Virtual DOM (Diffing Algorithm)
React then compares the previous and new Virtual DOM versions to identify exactly what has changed. This process is known as diffing.
- If an element remains the same, React keeps it as is
- If an element changes, React updates only the affected properties
- If an element is removed, React deletes it from the real DOM
- If a new element is added, React inserts it without disturbing existing nodes
React Efficiently Updates the Real DOM
Once the smallest possible set of changes is identified, React applies them to the real DOM in the most optimized way possible.
This prevents unnecessary layout recalculations and reflows, ensuring smooth performance even with frequent updates.
How React’s Reconciliation Optimizes Performance
The Diffing Algorithm
React’s diffing algorithm enables intelligent updates instead of blindly re-rendering everything. It follows three key rules:
- Elements of the same type are compared → If a
<div>
remains a<div>
, React updates only its properties instead of replacing it - Elements of different types are replaced → If a
<p>
is changed to an<h1>
, React removes the old element and inserts a new one - Keys in lists help track changes efficiently → When rendering lists, React uses keys to avoid unnecessary re-renders and rearrange elements properly
Batching Updates to Reduce Renders
React batches multiple state updates into a single render cycle, preventing redundant re-renders.
For example, instead of processing these separately:
setCount(count + 1);
setName("Sunil Sharma");
setActive(true);
React batches them together into a single optimized update, reducing the number of times the UI re-renders.
Optimized List Rendering with Keys
React uses keys to track elements efficiently when rendering lists. Without proper keys, React may re-render entire lists unnecessarily, reducing performance.
By assigning unique, stable keys, React ensures that:
- Items are correctly mapped between renders
- Only modified elements are updated
- The UI remains stable even when items are added, removed, or moved
How Reconciliation Works with React’s Fiber Architecture
The Evolution of React Fiber
React’s Fiber Architecture, introduced in React 16, fundamentally changed how reconciliation works by making rendering asynchronous and interruptible.
Previously, React followed a synchronous rendering model, meaning all updates had to be processed in one go, which could block the main thread and cause UI lag.
With Fiber, React now splits rendering work into smaller units that can be paused, resumed, or reprioritized based on user interactions.
Benefits of Fiber in Reconciliation
- Prioritization of important updates → User interactions (like typing or clicking) are prioritized over background updates
- Smooth animations and transitions → Long-running UI updates are split into manageable chunks
- Support for concurrent rendering → Multiple UI updates can be processed in parallel
This makes reconciliation even more efficient because React can now schedule updates intelligently based on performance needs.
React 19: Advancements in Reconciliation
React 19 introduces even smarter optimizations to make reconciliation more seamless.
Automatic Memoization
Previously, developers had to use useMemo
and useCallback
to optimize performance manually. React 19 now automatically memoizes functions and values, reducing unnecessary calculations.
Smarter Diffing and Reconciliation
React 19 improves reconciliation with:
- Better tracking of UI changes to avoid unnecessary renders
- Optimized list reconciliation for dynamic content
- More efficient handling of large state updates
Automatic Batching of State Updates
React 19 ensures that multiple state updates happening within the same event loop are automatically batched, preventing extra renders and improving UI responsiveness.
Real-World Example: How Reconciliation Improves Performance
Consider a real-time stock market dashboard where hundreds of stock prices update every second.
Without Reconciliation
- Every price change triggers a full re-render of the stock list
- The entire UI slows down as React processes excessive updates
With Reconciliation
- React updates only the modified stock prices, leaving the rest of the UI untouched
- Performance remains fast and efficient, even with frequent updates
This demonstrates why reconciliation is critical for performance-heavy applications.
Reconciliation in React vs Other Frameworks
React vs Vue
- Vue’s Virtual DOM also uses diffing, but Vue tracks dependencies more explicitly through its reactive system
- React’s Fiber allows asynchronous rendering, while Vue’s Virtual DOM updates synchronously
React vs Svelte
- Svelte compiles components into optimized JavaScript, eliminating the need for a Virtual DOM altogether
- React uses reconciliation to efficiently update UI, while Svelte re-generates minimal changes at build time
While Svelte aims to remove the need for a Virtual DOM, React’s reconciliation is optimized for large-scale, dynamic applications, making it more flexible for complex UIs.
Final Thoughts
Reconciliation is at the core of React’s performance-first architecture. By leveraging:
- Virtual DOM diffing
- Batching updates
- Optimized list rendering
- Fiber’s concurrent rendering
- React 19’s automatic memoization
React ensures efficient UI updates while keeping applications smooth and responsive.
Key Takeaway
If you understand how React processes updates, you can avoid unnecessary renders, write more efficient components, and build high-performance applications.
What are your thoughts on reconciliation? Have you encountered performance challenges in your React projects?