Table Of Contents
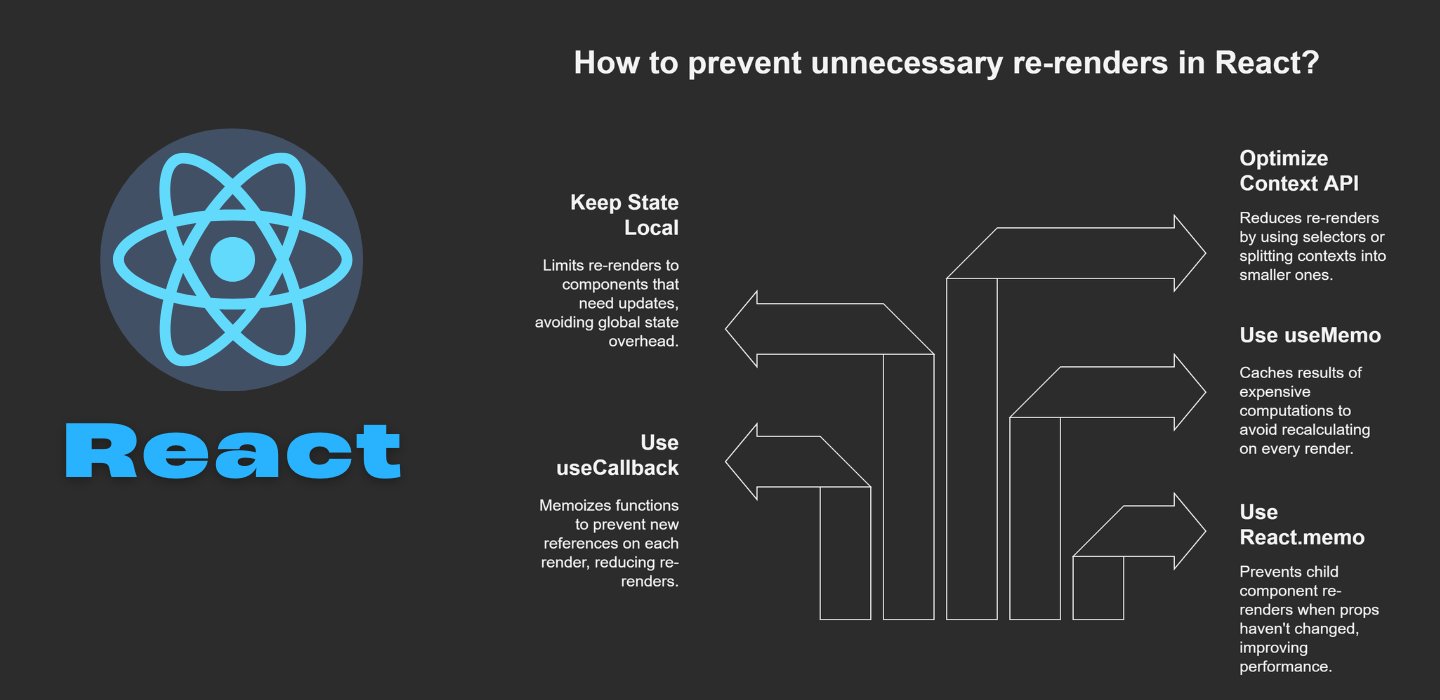
Preventing Unnecessary Re-Renders in React and Why It Matters
Performance optimization is one of the most critical aspects of React development. A well-structured React application should feel fast, responsive, and efficient. However, one of the biggest performance bottlenecks developers face is unnecessary re-renders. If components re-render when they do not need to, the application slows down, leading to poor user experience and increased computational load.
Preventing unnecessary re-renders is not just about writing better code, but also about understanding how React works under the hood.
Why Do Unnecessary Re-Renders Happen?
React’s rendering behavior is based on state changes, props updates, and context changes. Whenever any of these factors change, React re-renders the affected component and its child components. However, in many cases, re-renders happen even when the output remains the same, consuming unnecessary processing power.
The most common causes of unnecessary re-renders include:
- Parent Component Re-Renders: If a parent component re-renders, all child components inside it will also re-render, even if their props have not changed.
- New Object and Function References: Every time a component renders, new objects or functions get created, causing child components to think props have changed.
- State Updates That Do Not Change Values: If a state update is triggered but the new value is identical to the previous one, React will still re-render the component.
- Context API Re-Renders: When a context value updates, every component consuming that context re-renders, even if it does not use the changed value.
Understanding these causes is the first step toward preventing unnecessary re-renders and optimizing performance.
How to Prevent Unnecessary Re-Renders in React?
1. Use React.memo to Prevent Child Component Re-Renders
If a child component receives the same props repeatedly, React will still re-render it unless it is explicitly memoized. React.memo prevents unnecessary re-renders by only re-rendering the component when its props change.
This is particularly useful for components that:
- Receive static props that do not change often
- Perform expensive computations
- Render large amounts of UI elements
Using React.memo helps reduce the rendering workload and improves UI responsiveness.
2. Use useCallback to Memoize Functions Passed as Props
Functions are reference types, meaning a new function is created every time a component re-renders. This leads to child components thinking that their props have changed, causing unnecessary re-renders.
To prevent this, use useCallback to memoize functions and ensure that they only get recreated when dependencies change.
When to Use useCallback?
- When passing functions to child components wrapped in React.memo
- When handling event listeners inside child components
- When defining callback functions that do not change frequently
3. Use useMemo for Expensive Computations
Some components perform heavy calculations based on props or state. If these calculations run on every re-render, they can significantly slow down the application.
The useMemo hook caches the result of an expensive computation, ensuring that the function only re-runs if its dependencies change.
When to Use useMemo?
- When performing expensive mathematical operations
- When filtering or transforming large datasets
- When computing derived values from props or state
Using useMemo reduces the computational load and keeps the UI fast and responsive.
4. Keep State Local When Possible
Global state updates trigger more re-renders than necessary. If a piece of state only affects a specific part of the UI, keeping it local to that component prevents unnecessary re-renders in unrelated components.
How to Optimize State Management?
- Avoid keeping everything in a global state like Redux or Context if it is not needed globally.
- Lift state only when necessary, ensuring minimal re-renders across the component tree.
- Prefer component-level state when a piece of data only concerns a specific component.
By keeping state local, you ensure that only the components that need updates get re-rendered, rather than the entire application.
5. Optimize Context API Usage
React’s Context API is a powerful tool for prop drilling elimination, but it comes with a major downside—whenever a context value changes, all consuming components re-render.
To prevent unnecessary re-renders caused by Context updates:
- Use Context selectors instead of consuming the entire context
- Split large contexts into smaller, more specific ones
- Use memoization techniques to avoid frequent updates
By structuring Context efficiently, you reduce unnecessary component updates and improve app performance.
6. Avoid Updating the State with the Same Value
React does not compare state updates deeply—if a state update is triggered with the same value, React still triggers a re-render.
Example of Avoiding Unnecessary State Updates:
- Check if the new state is different before setting it
- Use functional updates to ensure state updates are meaningful
- Avoid unnecessary API calls or data mutations that do not impact the UI
This helps React skip unnecessary re-renders and optimizes the app.
7. Profile and Debug Re-Renders Using React DevTools
Even with best practices, unnecessary re-renders can still happen. React DevTools Profiler allows developers to:
- Track which components re-render unnecessarily
- Identify slow rendering operations
- Optimize state and props flow for better efficiency
Using React DevTools to visualize rendering behavior helps developers make targeted performance improvements.
Why Preventing Unnecessary Re-Renders Matters?
React applications should feel smooth and responsive, but frequent and unnecessary re-renders can lead to:
- Increased CPU and memory usage
- Laggy UI updates and slow animations
- Higher power consumption on mobile devices
- Poor user experience in complex applications
By optimizing rendering behavior, developers improve application efficiency, reduce computational overhead, and deliver a better experience to users.
Final Thoughts
Preventing unnecessary re-renders is not just about improving speed—it is about writing efficient, scalable, and well-structured React applications.
- React.memo stops unnecessary child component re-renders
- useCallback prevents function recreation in props
- useMemo optimizes expensive calculations
- Keeping state local prevents global re-renders
- Optimizing Context API ensures minimal re-rendering overhead
When React apps re-render efficiently, the entire application feels smoother, more performant, and optimized for real-world use cases.
The Question
What is the most common re-render issue you have faced in React? Have you used memoization techniques to improve performance?