TUTORIAL PLAYLIST
6-Month Internship Guide: Path to Your Dream Job in 2025
Create a Vision BoardSecuring Full-Stack Engineering Roles in Tier 1, Tier 2, and Top-Level MNCs
Your Journey to a Dream JobAlpha Web Development Standards 2025
Full-Stack Mastery BlueprintJavaScript Basics – Environment Setup & Introduction for Beginners
Session 01JavaScript Fundamentals Step-by-Step
Session 02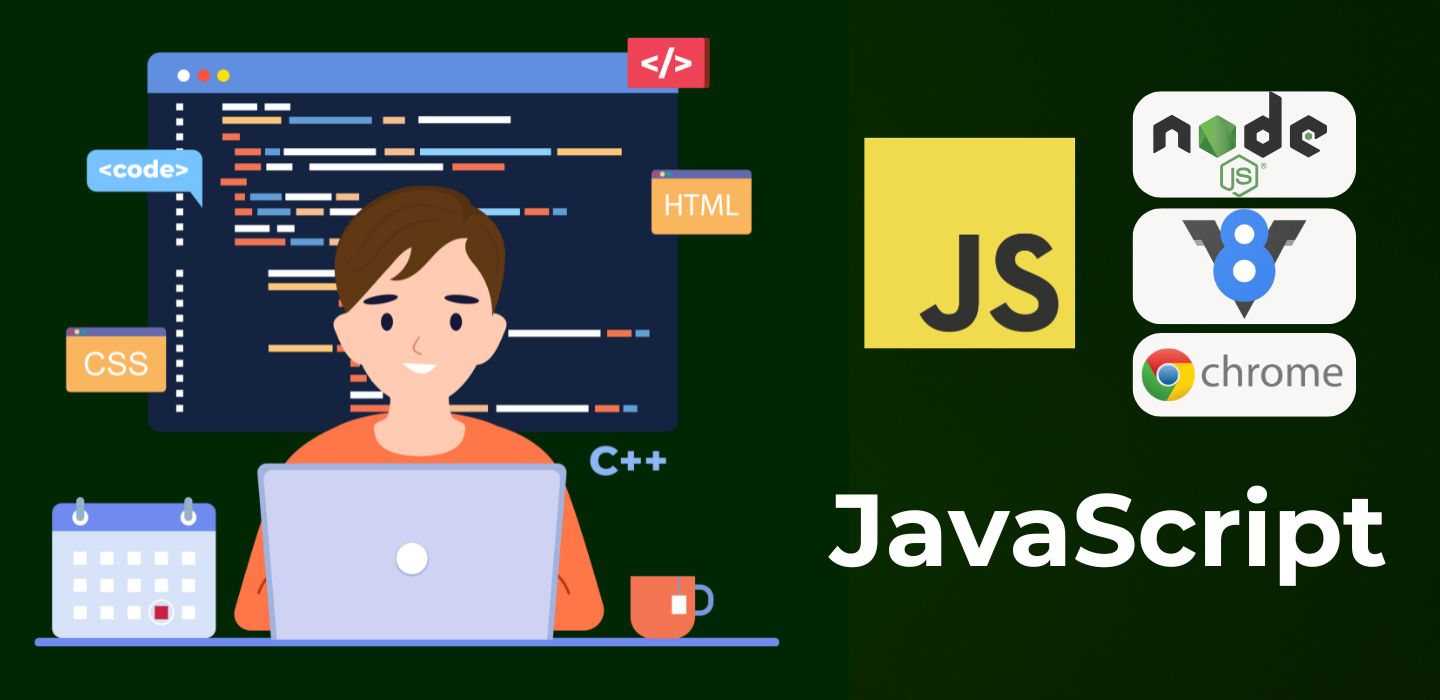
Environment Setup and Introduction for New Developers
JavaScript is the foundation of modern web development, powering everything from interactive websites to powerful backend applications. Whether you’re looking to build stunning front-end interfaces, dynamic web applications, or even full-stack solutions, mastering JavaScript is your first step toward success.
This session is designed to give you a solid start by introducing you to JavaScript, its evolution, and why it remains the most in-demand programming language today. You’ll learn how to set up a professional development environment, install Node.js (LTS version) for running JavaScript outside the browser, and write your first JavaScript program.
By the end of this session, you’ll not only understand JavaScript’s significance but also have a fully functional setup to begin coding confidently. Let’s build a strong foundation together because great developers start with great fundamentals.
Table of Contents
- Introduction to JavaScript
- Evolution and History of JavaScript
- Setting Up a JavaScript Development Environment
- Writing and Running Your First JavaScript Program
- Key Theoretical Concepts Behind JavaScript Execution
- Best Practices for JavaScript Development
- Summary of the Session
- Next Steps & Homework
Introduction to JavaScript
What is JavaScript?
JavaScript is a high-level, dynamically typed, interpreted programming language designed for web development. It enables developers to create interactive, dynamic, and feature-rich web applications by manipulating HTML, CSS, and other browser-based technologies.
Unlike other languages that require compilation, JavaScript is executed directly in the browser by a JavaScript engine. This means developers can write code and see immediate results without needing complex compilation steps.
Evolution and History of JavaScript
JavaScript was created in 1995 by Brendan Eich while working at Netscape. Initially, it was called Mocha, then LiveScript, before finally being renamed JavaScript.
Over time, JavaScript has undergone significant improvements with the introduction of the ECMAScript (ES) standards, which define how JavaScript should function.
Some major milestones in JavaScript history:
- 1995: JavaScript was created for Netscape Navigator.
- 1997: ECMAScript (ES1) was released, standardizing JavaScript.
- 2009: ES5 introduced modern features like JSON support and strict mode.
- 2015 (ES6/ES2015): A revolutionary update that introduced
let
andconst
, arrow functions, template literals, and modules. - 2020-Present: JavaScript continues to evolve with features like optional chaining, private class fields, and better async handling.
Setting Up a JavaScript Development Environment
A proper development environment ensures smooth coding, debugging, and execution. We will set up everything step by step.
Installing a Code Editor
For JavaScript development, a professional code editor is essential. The recommended editor is Visual Studio Code (VS Code) because of its lightweight nature, built-in debugging tools, and extensive plugin support.
Steps to Install VS Code
- Download VS Code from https://code.visualstudio.com.
- Install it on your system following the on-screen instructions.
- Open VS Code and install the following extensions to enhance your JavaScript workflow:
- ESLint: Helps maintain clean and consistent code formatting.
- JavaScript (ES6) Code Snippets: Provides useful code templates for faster coding.
- Live Server: Allows real-time reloading of your code changes in the browser.
Installing Node.js (Understanding LTS vs. Current Versions)
Why Install Node.js?
Node.js allows JavaScript to run outside the browser, making it useful for building backend applications, running scripts, and using modern JavaScript tooling.
Understanding Node.js LTS vs. Current Versions
- LTS (Long-Term Support):
- More stable, recommended for production environments.
- Receives security patches and bug fixes for an extended period.
- Suitable for most developers who need reliability.
- Example: Node.js 18.x LTS.
- Current Version:
- Includes the latest features but might be unstable.
- Good for testing new JavaScript capabilities.
- Not recommended for production use.
- Example: Node.js 20.x (Current).
For this session, we will install Node.js LTS, which ensures stability.
Steps to Install Node.js LTS
- Download Node.js LTS version from https://nodejs.org.
- Install it using the default settings.
- Verify the installation:
node -v # Displays Node.js version
npm -v # Displays npm (Node Package Manager) version
If these commands return version numbers, Node.js and npm are correctly installed.
Setting Up a JavaScript Project
To create a proper workspace for writing JavaScript code:
- Open the terminal (Command Prompt or VS Code terminal).
- Create a project folder and navigate into it:
mkdir js-basics && cd js-basics
- Initialize a JavaScript project (Optional for now):
npm init -y
This creates a package.json
file, which helps in managing project dependencies later.
Writing and Running Your First JavaScript Program
Writing a Simple “Hello, World” Program
We will create a file named hello.js
and write a basic JavaScript script.
Code (hello.js)
// This is a simple JavaScript program that prints a message to the console
// Using console.log() to output text to the terminal
console.log("Welcome to SunilSharmaTechie’s JavaScript Tutorial!");
Running the Program
Option 1: Running JavaScript in Node.js (Terminal Method)
- Open VS Code and navigate to your project directory.
- Run the script using Node.js:
node hello.js
- Expected output:
Welcome to SunilSharmaTechie’s JavaScript Tutorial!
Option 2: Running JavaScript in the Browser Console
- Open Google Chrome or any modern browser.
- Right-click and select Inspect, then go to the Console tab.
- Type the following command and press Enter:
console.log("Welcome to SunilSharmaTechie’s JavaScript Tutorial!");
- You will see the message printed in the console.
Key Theoretical Concepts Behind JavaScript Execution
JavaScript is an Interpreted Language
JavaScript executes code line by line using a JavaScript engine such as V8 (Chrome), SpiderMonkey (Firefox), or JavaScriptCore (Safari).
Understanding JavaScript Execution
- Browser Execution: JavaScript runs inside a webpage to manipulate elements dynamically.
- Node.js Execution: JavaScript runs as a standalone program outside the browser.
Best Practices for JavaScript Development
- Confirm Your Environment Setup
- Always check that Node.js and npm are installed before running any JavaScript code.
- Follow Proper Code Formatting
- Use indentation, comments, and meaningful variable names for better readability.
- Use Debugging Tools
- The
console.log()
function helps debug values and outputs. - Use the
debugger;
statement to pause execution in the browser.
- The
Summary of the Session
- JavaScript is the backbone of web development and runs both on the frontend and backend.
- We installed VS Code and Node.js LTS to set up a professional coding environment.
- We wrote and executed our first JavaScript program.
- We explored how JavaScript executes in different environments.
Next Steps & Homework
- Write a JavaScript script that prints your name and your favorite programming language.
- Run it using both Node.js and the browser console.
- Explore VS Code extensions and test different debugging techniques.
Coming up next: JavaScript Fundamentals – Variables, Data Types, and Operators.