Table Of Contents
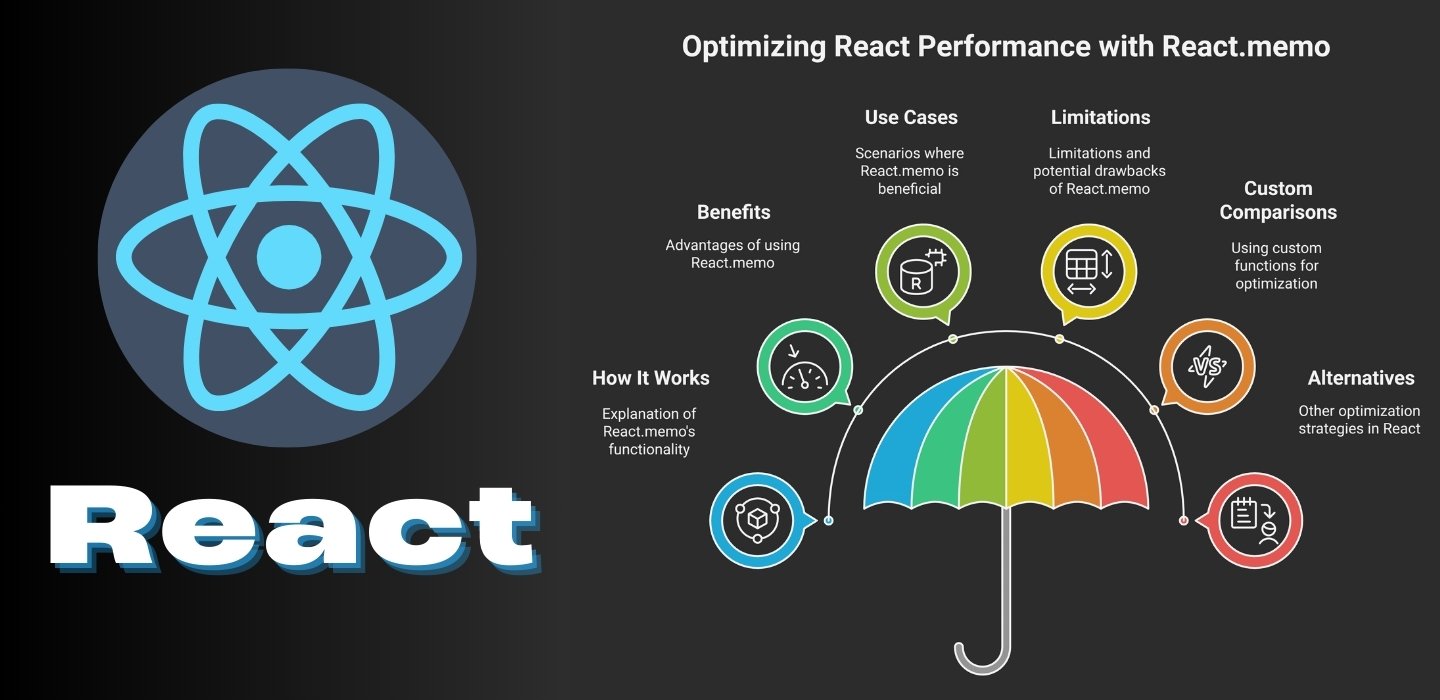
Understanding React.memo and Why It Matters
When developing React applications, performance optimization is crucial, especially for large-scale projects or applications with complex UI updates. One of the most effective yet often overlooked tools for optimizing performance in React is React.memo
React.memo is a higher-order component that helps prevent unnecessary re-renders in functional components. It memoizes the component’s output and only re-renders it when its props change. This reduces computations and improves efficiency, making React applications faster and more responsive
How React.memo Works
The syntax for React.memo is simple and easy to implement
import React from 'react'
const MyComponent = React.memo(function MyComponent({ name }) {
console.log('Component rendered!')
return <div>Hello, {name}</div>
})
When this component is used, React checks if the name
prop has changed. If it has not, React.memo prevents the component from re-rendering and reuses the previously rendered output
Breaking It Down
React.memo wraps a functional component and remembers the last rendered result
If the props do not change, React skips rendering and reuses the previous output
If the props do change, React triggers a re-render as usual
This approach is particularly useful in components that receive the same props frequently but do not require updates unless the data changes
Where Should You Use React.memo?
React.memo should not be applied to every component, but it is particularly beneficial in specific scenarios
Components That Re-Render Frequently
If a component is getting re-rendered unnecessarily due to changes in its parent component, React.memo can help reduce those unnecessary updates
Lists and Large Datasets
When rendering large lists of items, such as tables, dashboards, or product catalogs, preventing unnecessary re-renders can significantly improve performance
Components with Expensive Calculations
If a component performs complex calculations, such as filtering or sorting large datasets, memoization helps avoid unnecessary processing
Preventing Unwanted UI Updates
Interactive components such as dashboards, notifications, and dynamic widgets that update frequently but do not need to be re-rendered every time benefit from React.memo
When Not to Use React.memo
While React.memo is a great optimization tool, overusing it can sometimes increase memory consumption and make debugging more complex
Avoid using React.memo in these cases
If the component always receives different props, React.memo will not provide any benefits
If the component is lightweight and renders quickly, memoization adds unnecessary overhead
If the component relies on local state that changes frequently, React.memo will not optimize performance effectively
If the application uses context values, which change frequently, memoization will not be effective unless combined with useMemo
or useCallback
Enhancing React.memo with Custom Comparison Functions
By default, React.memo performs a shallow comparison of props. However, if a component receives nested objects, it might still re-render even when the values inside those objects do not change
To optimize further, use a custom comparison function
const MyComponent = React.memo(
function MyComponent({ user }) {
console.log('Component rendered!')
return <div>{user.name}</div>
},
(prevProps, nextProps) => {
return prevProps.user.id === nextProps.user.id
}
)
In this case, the component will only re-render if the user.id
value changes, preventing unnecessary updates when other unrelated properties change
Alternatives to React.memo for Performance Optimization
If React.memo alone is not improving performance, consider other optimization strategies
useMemo
– Memoizes computed values to prevent re-computationuseCallback
– Memoizes function instances to prevent unnecessary re-creation
Optimizing dependencies in useEffect
– Reducing unnecessary re-execution of effects
How React.memo Improves Performance in Real Applications
E-commerce websites prevent unnecessary re-renders in product listings
Financial dashboards improve efficiency in handling real-time data
AI-driven applications optimize the rendering of interactive visualizations
Streaming platforms enhance performance when handling dynamic user interfaces
Final Thoughts on React.memo
React.memo is an effective tool for preventing unnecessary re-renders in functional components, but it should be used strategically
If a component re-renders unnecessarily, use React.memo to reduce performance overhead
If the component is small and lightweight, avoid memoization unless necessary
If props contain nested objects, use a custom comparison function to ensure better optimization
Always profile performance before and after using React.memo to measure the actual impact
React.memo is not a silver bullet, but when used correctly, it can significantly improve React application performance without compromising scalability
Would you implement React.memo in your next project?